有关数组的一些方法
- Array.isArray(ary) //判断变量是不是一个数组,返回布尔值
- toString() // 把数组转换为字符串,并返回结果,结果使用逗号分隔
var ary = ['a', 'b', 'c'];
var ary1 = [1, 2, 3];
console.log(ary.toString());
console.log(ary1.toString());
控制台的输出结果如下:

3. valueOf() // 返回数组对象本身
var ary = ['a', 'b', 'c'];
console.log(ary.valueOf());
- ary.pop() [常用] //删除并返回数组的最后一个元素
var ary = ['a', 'b', 'c'];
console.log(ary.pop());
console.log(ary);
控制台的输出结果如下:
5. ary.shift() //删除并返回数组的第一个元素
var ary = ['a', 'b', 'c'];
console.log(ary.shift());
console.log(ary);
控制台的输出结果如下:
6. ary.push(要添加的元素1,要添加的元素2,…,要添加的元素n )[常用] // 向数组的末尾添加一个或多个元素,并返回新的长度。
var ary = ['a', 'b', 'c'];
console.log(ary.push('d', 'e'));
console.log(ary);
控制台的输出结果如下:
7. ary.unshift(要添加的元素1,要添加的元素2,…,要添加的元素n) //向数组的开头添加一个或更多元素,并返回新的长度。
var ary = ['a', 'b', 'c'];
console.log(ary.unshift('d', 'e'));
console.log(ary);
控制台的输出结果如下:
8. reverse() // 翻转数组
var ary = ['a', 'b', 'c'];
console.log(ary.reverse());
控制台的输出结果如下:
[注]: 该方法会改变原来的数组,而不会创建新的数组
9. concat() //连接两个或更多的数组,返回一个新数组,不改变原数组。
var ary = ['a', 'b', 'c'];
var ary1 = ['d', 'e', 'f'];
// 添加元素
console.log(ary.concat('d', 'e'));
// 连接数组
console.log(ary.concat(ary1));
console.log(ary);
控制台的输出结果如下:
10. slice(startindex, endindex) //从当前数组中截取一个新的数组 ,返回选定的元素
✔第一个参数表示开始索引位置,如果是负数,那么它规定从数组尾部开始算起的位置。也就是说,-1 指最后一个元素,-2 指倒数第二个元素,以此类推。
✔第二个参数代表结束索引位置,如果没有指定该参数,那么切分的数组包含从 start 到数组结束的所有元素。
var ary = ["a", "b", "c", 'd', 'e'];
console.log(ary.slice(1));
console.log(ary.slice(1,3));
console.log(ary);
控制台的输出结果如下:
[注]:截取的元素不包含结束索引位置的元素。
11. splice(startindex, deletCont, options) //从数组中添加或删除元素,然后返回被删除的元素的集合
✔ 第一个参数代表添加/删除元素的位置,使用负数可从数组结尾处规定位置。
✔ 第二个参数代表要删除的元素数量。如果设置为 0,则不会删除元素。
✔ 第三个参数代表向数组插入的新元素
var ary = ["a", "b", "c", "d", "e"];
// 从索引为1的位置开始添加元素f,g,h
console.log(ary.splice(1, 0, "f",'g','h'));
console.log(ary);
// 从索引为6的位置开始添加元素g
console.log(ary.splice(6, 0, "g"));
console.log(ary);
// 删除从索引为2的位置开始的3个元素
console.log(ary.splice(2, 3));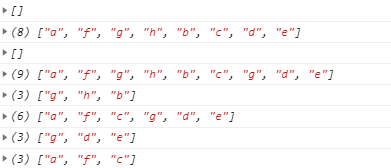
console.log(ary);
// 删除从索引为3的位置开始的所有元素
console.log(ary.splice(3));
console.log(ary);
控制台的输出结果如下:
12. indexOf(content[,index]),lastIndexOf() //没找到返回-1
找数组中的某个值
✔ indexOf():如果找到返回元素的第一个索引位置,如果没有找到返回-1
✔ lastIndexOf():如果找到返回元素的最后一个索引位置,如果没有找到返回-1
var ary = ["a", "b", "b","c", "d", "b","e"];
// 添加元素f
console.log(ary.indexOf('b'));
console.log(ary.indexOf('bc'));
console.log(ary.lastIndexOf('b'));
控制台的输出结果如下:
13. join() // 将数组中的每一个元素通过一个字符连接到一起
var ary = ['张三', '李四', '王五'];
console.log( ary.join('|') );
console.log( ary.join('-') );
控制台的输出结果如下:
ES5新增方法
forEach(function(value, index, array){}):遍历数组
回调函数的参数
value:数组元素 ,必写
index:数组元素的索引,可选
array:当前的数组,可选
[注]:没有返回值
// forEach 迭代(遍历) 数组
var arr = [1, 2, 3];
var sum = 0;
arr.forEach(function(value, index, array) {
console.log('每个数组元素' + value);
console.log('每个数组元素的索引号' + index);
console.log('数组本身' + array);
sum += value;
})
console.log(sum);
控制台的输出结果如下:
filter(function(value, index,array){}):过滤数组
回调函数的参数
value:数组元素
index:数组元素的索引
array:当前的数组
返回值是一个新数组
// filter 筛选数组
var arr = [12, 66, 4, 88, 3, 7];
var newArr = arr.filter(function(value, index) {
return value >= 20;
});
console.log(newArr);
以数组形式输出大于20的元素:
some(function(value, index,array),thisValue):查找数组中是否有满足条件的元素
回调函数的参数
value:数组元素
index:数组元素的索引
array:当前的数组
返回值
如果找到满足条件的元素,则返回true,否则返回false
var arr = [10, 30, 4];
var flag = arr.some(function(value) {
return value >= 20;
});
console.log(flag);
数组是否有大于20的值:
[总结]:1. filter 用于查找满足条件的元素,返回的是一个数组,会把所有满足条件的元素返回回来。
2. some 用于查找满足条件的元素是否存在 ,返回的是一个布尔值,如果查找到第一个满足条件的元素就终止循环。
map(function(currentValue,index,arr), thisValue)
回调函数的参数
currentValue:数组元素
index:数组元素的索引
arr:当前的数组
返回值
返回一个新数组,数组中的元素为原始数组元素调用函数处理后的值。
var ary = [4, 9, 16, 25];
var newAry = ary.map(function(value, index){
return Math.sqrt(value);
});
console.log(newAry);
对数组中的每个元素开平方后输出:
[总结]:forEach和map的比较:
- forEach:遍历数组,没有返回值
- map:遍历数组,会返回一个新数组
数组的扩展方法(ES6)
扩展运算符(展开语法)
- 扩展运算符可以将数组或者对象转为用逗号分隔的参数序列
var ary = ["a", "b", "c"];
var ary1 = [1 ,2 ,3];
console.log(...ary); //相当于下面一行代码
console.log("a", "b", "c")
console.log(...ary1);
控制台的输出结果如下:
[注]:从控制台输出的结果来看,…ary相当于把数组中的元素取出来
2. 扩展运算符可以应用于合并数组
// 方法一
var ary1 = [1, 2, 3];
var ary2 = [3, 4, 5];
var ary3 = [...ary1, ...ary2];
console.log(ary3);
// 方法二
ary1.push(...ary2);
console.log(ary1);
合并ary1,ary2:
3. 将伪数组或可遍历对象转换为真正的数组
var oDivs = document.getElementsByTagName('div');
oDivs = [...oDivs];
console.log(oDivs);
将伪数组转换为真正的数组,可使用数组的方法:
构造函数方法:Array.from()
- 将伪数组或可遍历对象转换为真正的数组
var arrayLike = {
"0": "a",
"1": "b",
"2": "c",
length: 3
}; //转成数组
var arr2 = Array.from(arrayLike); // ['a', 'b', 'c']
console.log(arr2);
将对象转成数组:
2. 方法还可以接受第二个参数,作用类似于数组的map方法,用来对每个元素进行处理, 将处理后的值放入返回的数组
var arrayLike = {
"0": 1,
"1": 2,
length: 2
};
var newAry = Array.from(arrayLike, function(item){
return item * 2;
});
console.log(newAry);
传第二个参数(函数),让数组的每个元素*2:
[注]:如果是对象,那么属性需要写对应的索引,且要通过属性length指定数组的长度。
实例方法:find(function(currentValue, index,arr))
回调函数的参数
currentValue:当前遍历的元素,必写
index:当前元素的索引,可选
arr:当前元素所在的数组对象,可选
返回值
返回符合条件第一个数组成员,如果没有找到则返回undefined
var ary = [
{
id: 1,
name: "张三",
},
{
id: 2,
name: "李四",
},
{
id: 3,
name: "李四",
},
];
var target = ary.find(function(item, index){
return item.name == '李四';
});
console.log(target);
查找name为李四的数组元素:
实例方法:findIndex(function(currentValue, index, arr), thisValue)
参数
-
function():
currentValue:当前元素,必写
index:当前元素的索引,可选
arr:当前元素所在的数组对象,可选
-
thisValue:可选。 传递给函数的值一般用 “this” 值。
如果这个参数为空, “undefined” 会传递给 “this” 值
返回值
返回第一个符合条件的数组成员的位置,如果没有找到返回-1
var ary = [1, 5, 10, 15];
var index= ary.findIndex(function(value, index){
return value > 9;
});
console.log(index);
输出数组中大于9的值所在的位置(索引):
实例方法:includes(searchElement,fromIndex):判断某个数组是否包含给定的值
参数
searchElement:必须。需要查找的元素值。
fromIndex:可选。从该索引处开始查找 searchElement。如果为负值,则按升序从 array.length + fromIndex 的索引开始搜索。默认为 0。
返回值
布尔值,找到返回true,否则返回false
var item1 = [1, 2, 3].includes(2);
var item2 = [1, 2, 3].includes(4);
// 从索引为2的位置开始往右查找1
var item3 = [1, 2, 3, 4].includes(1, 2);
// 从索引为2(4-2)的位置开始往右查找3
var item4 = [1, 2, 3, 4].includes(3, -2);
// 从索引为2(4-2)的位置开始往右查找2
var item5 = [1, 2, 3, 4].includes(2, -2);
console.log(item1);
console.log(item2);
console.log(item3);
console.log(item4);
console.log(item5);
控制台输出结果:
[参考文档]:
[1] w3school:https://www.w3school.com.cn/
[2] 菜鸟教程:https://www.runoob.com/