GD32F303的RTC功能(包括閏年平年的年月日星期時分秒)(時間設定和擷取正确,适用于使用32位的計數器的MCU)
針對RTC的設定和擷取,應該測試一些特殊值如:閏年的最後一天2020.12.31,閏年的2月28.29,平年的2月28,1月30,7月30.31,8月30.31等,如果這些日期沒有問題,基本确定是沒有問題的;
以下為我的代碼,已測試全部,沒有問題,除了上面日期,還包括測試這些上面日期在(1)設定後斷電後,等到下一日再上電時間;(2)設定後斷電後,未到下一日的再上電時間;(3)設定後斷電,等到過了第二日再上電時間;功能全部正常;
#include “gd32f30x.h”
#include “RTC.h”
#include “LCD.h”
//enter the second interruption,set the second interrupt flag to 1
volatile uint32_t timedisplay;
//月份資料表
uint8_t const table_week[12]={0,3,3,6,1,4,6,2,5,0,3,5}; //月修正資料表
//平年的月份日期表
const uint8_t mon_table[12]={31,28,31,30,31,30,31,31,30,31,30,31};
void RTC_Init(void)
{
if (bkp_read_data(BKP_DATA_0) != 0xA5A5){
//RTC configuration
rtc_configuration();
//adjust time by values entred by the user on the hyperterminal
//time_adjust();
RTC_Set(2011,01,01,0,0,0);//預設時間
bkp_write_data(BKP_DATA_0, 0xA5A5);
}else{
/* check if the power on reset flag is set */
if (rcu_flag_get(RCU_FLAG_PORRST) != RESET){
// printf("\r\n\n Power On Reset occurred....");
}else if (rcu_flag_get(RCU_FLAG_SWRST) != RESET){
/* check if the pin reset flag is set */
// printf("\r\n\n External Reset occurred....");
}
/* allow access to BKP domain */
rcu_periph_clock_enable(RCU_PMU);
pmu_backup_write_enable();
//printf("\r\n No need to configure RTC....");
/* wait for RTC registers synchronization */
rtc_register_sync_wait();
rtc_lwoff_wait();
/* enable the RTC second */
rtc_interrupt_enable(RTC_INT_SECOND);
//rtc_interrupt_enable(RTC_INT_ALARM);
/* wait until last write operation on RTC registers has finished */
rtc_lwoff_wait();
}
//clear reset flags
rcu_all_reset_flag_clear();
RTC_Get();
nvic_priority_group_set(NVIC_PRIGROUP_PRE1_SUB3);
nvic_irq_enable(RTC_IRQn,1,0);
}
//功能描述:判斷是否是閏年函數
//輸入:年份
//輸出:該年份是不是閏年.1:是, 0:不是
uint8_t Is_Leap_Year(uint16_t year)
{
if(((year%4==0)&&(year%100!=0))||((year%100==0)&&(year%400==0))){
return 1;
}else{
return 0;
}
}
//功能描述:設定時鐘
//把輸入的時鐘轉換為秒鐘
//以1970年1月1日為基準
//1970~2099為合法年份
//傳回值:0:成功,其他:錯誤
uint8_t RTC_Set(uint16_t syear,uint8_t smon,uint8_t sday,uint8_t hour,uint8_t min,uint8_t sec)
{
uint16_t t;
uint32_t seccount=0;
if(syear<2000||syear>2099)return 1;//syear範圍1970-2099,此處設定範圍為2000-2099
for(t=1970;t<syear;t++) //所有年份的秒鐘相加
{
if(Is_Leap_Year(t)){
seccount+=31622400;//閏年的秒鐘數
}else{
seccount+=31536000; //平年的秒鐘數
}
}
smon-=1;
for(t=0;t<smon;t++) //把前面月份的秒鐘數相加
{
seccount+=(uint32_t)mon_table[t]*86400;//月份秒鐘數相加
if((Is_Leap_Year(syear)) && (t==1)){
seccount+=86400;//閏年2月份增加一天的秒鐘數
}
}
seccount+=(uint32_t)(sday-1)*86400;//把前面日期的秒鐘數相加
seccount+=(uint32_t)hour*3600;//小時秒鐘數
seccount+=(uint32_t)min*60; //分鐘秒鐘數
seccount+=sec;//最後的秒鐘加上去
/* change the current time */
rtc_counter_set(seccount);
rtc_lwoff_wait();
return 0;
}
//得到目前的時間
//傳回值:0:成功,其他:錯誤
uint8_t RTC_Get(void)
{
static uint16_t daycnt=0;
uint32_t timecount=0;
uint32_t temp=0;
uint16_t temp1=0;
timecount=rtc_counter_get();
Current_Clock = timecount;
temp=timecount/86400;
if(daycnt!=temp)
{
daycnt=temp;
temp1=1970; //1970年開始
while(temp>=365)
{
if(Is_Leap_Year(temp1))//閏年
{
if(temp>=366){
temp-=366;//閏年的秒鐘數
}else {
break;
}
}
else{
temp-=365; //平年
}
temp1++;
}
Current_year=temp1;//得到年份
temp1=0;
while(temp>=28)
{
if(Is_Leap_Year(Current_year)&&temp1==1)
{
if(temp>=29){
temp-=29;//閏年的秒鐘數
}else{
break;
}
}
else
{
if(temp>=mon_table[temp1]){
temp-=mon_table[temp1];//平年
}else{
break;
}
}
temp1++;
}
Current_month=temp1+1;//得到月份
Current_date=temp+1; //得到日期
}
temp=timecount%86400; //得到秒鐘數
Current_hour=temp/3600; //小時
Current_min=(temp%3600)/60; //分鐘
Current_sec=(temp%3600)%60; //秒鐘
Current_week = CaculateWeekDay(Current_year,Current_month,Current_date)+1;
return 0;
}
uint8_t CaculateWeekDay(int y,int m,int d)
{
int week;
if((m==1)||(m==2)){
m+=12;
y--;
}
week=(d+2*m+3*(m+1)/5+y+y/4-y/100+y/400)%7;
return week;
}
pmu_backup_write_enable();
/* reset backup domain */
bkp_deinit();
/* enable LXTAL */
rcu_osci_on(RCU_LXTAL);
/* wait till LXTAL is ready */
rcu_osci_stab_wait(RCU_LXTAL);
/* select RCU_LXTAL as RTC clock source */
rcu_rtc_clock_config(RCU_RTCSRC_LXTAL);
/* enable RTC Clock */
rcu_periph_clock_enable(RCU_RTC);
/* wait for RTC registers synchronization */
rtc_register_sync_wait();
/* wait until last write operation on RTC registers has finished */
rtc_lwoff_wait();
/* enable the RTC second interrupt*/
rtc_interrupt_enable(RTC_INT_SECOND);
/* wait until last write operation on RTC registers has finished */
rtc_lwoff_wait();
/* set RTC prescaler: set RTC period to 1s */
rtc_prescaler_set(32767);
/* wait until last write operation on RTC registers has finished */
rtc_lwoff_wait();
}
/!
\brief adjust time
\param[in] none
\param[out] none
\retval none
/
void time_adjust(void)
{
uint32_t temp = 0;
/ wait until last write operation on RTC registers has finished /
rtc_lwoff_wait();
//temp = time_regulate();
temp = 2131104000+52592000+2886400+93600 + 4660 + 33;
/ change the current time /
rtc_counter_set(temp);
rtc_lwoff_wait();
/ set the alarm time = currenttime + 10 second*/
/* wait until last write operation on RTC registers has finished */
rtc_lwoff_wait();
}
void time_display(uint32_t timevar)
{
uint32_t thh = 0, tmm = 0, tss = 0;
/*Current_year = (timevar / 31104000);
Current_month = ((timevar % 31104000) / 2592000);
Current_date = ((timevar % 2592000) / 86400);
Current_hour = (timevar % 86400) / 3600;
Current_min = (timevar % 3600) / 60;
Current_sec = (timevar % 3600) % 60;
Current_week = CaculateWeekDay(Current_year+2000,Current_month,Current_date)+1;
*/
/* compute hours */
thh = (timevar % 86400) / 3600;
/* compute minutes */
tmm = (timevar % 3600) / 60;
/* compute seconds */
tss = (timevar % 3600) % 60;
//printf(" Time: %0.2d:%0.2d:%0.2d\r\n", thh, tmm, tss);
if((PageScrnPoint==0x01)&&(!Display_Watch_Logo)){
Watch_Show_Time(thh,tmm,tss);
}
}
void time_show(void)
{
//printf("\n\r");
/* infinite loop */
while (1){
/* if 1s has paased */
if (timedisplay == 1){
/* display current time */
Current_Clock=rtc_counter_get();
//temp = 5*31104000+12*2592000+30*86400+23*3600 + 59*60 + 3;
Current_year = Current_Clock / 31104000;
Current_month = (Current_Clock % 31104000) / 2592000;
Current_date = (Current_Clock % 2592000) / 86400;
Current_hour = (Current_Clock % 86400) / 3600;
Current_min = (Current_Clock % 3600) / 60;
Current_sec = (Current_Clock % 3600) % 60;
time_display(Current_Clock);
timedisplay = 0;
}
}
}
/!
\brief this function handles RTC global interrupt request
\param[in] none
\param[out] none
\retval none
/
void RTC_IRQHandler(void)
{
if (rtc_flag_get(RTC_FLAG_SECOND) != RESET){
/ clear the RTC second interrupt flag/
rtc_flag_clear(RTC_FLAG_SECOND);
timedisplay = 1;
Current_year_old=Current_year;
Current_month_old=Current_month;
Current_date_old=Current_date;
//Current_Clock=rtc_counter_get();
RTC_Get();
timer_disable(TIMER1);
nvic_irq_disable(EXTI5_9_IRQn);
time_display(Current_Clock);
timer_enable(TIMER1);
nvic_irq_enable(EXTI5_9_IRQn, 0U, 0U);
if((Current_hour==0)&&(Current_min==0)&&(Current_sec==0)){
//watch_set_date(Current_year+2000,Current_month,Current_date,Current_week);
watch_set_date(Current_year,Current_month,Current_date,Current_week);
}
}
}
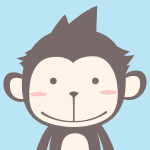
帶尺寸的圖檔:
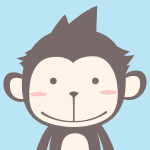
居中的圖檔:
居中并且帶尺寸的圖檔:
當然,我們為了讓使用者更加便捷,我們增加了圖檔拖拽功能。
如何插入一段漂亮的代碼片
去部落格設定頁面,選擇一款你喜歡的代碼片高亮樣式,下面展示同樣高亮的
代碼片
.
// An highlighted block
var foo = 'bar';
生成一個适合你的清單
- 項目
- 項目
- 項目
- 項目
- 項目1
- 項目2
- 項目3
- 計劃任務
- 完成任務
建立一個表格
一個簡單的表格是這麼建立的:
項目 | Value |
---|---|
電腦 | $1600 |
手機 | $12 |
導管 | $1 |
設定内容居中、居左、居右
使用
:---------:
居中
使用
:----------
居左
使用
----------:
居右
第一列 | 第二列 | 第三列 |
---|---|---|
第一列文本居中 | 第二列文本居右 | 第三列文本居左 |
SmartyPants
SmartyPants将ASCII标點字元轉換為“智能”印刷标點HTML實體。例如:
TYPE | ASCII | HTML |
---|---|---|
Single backticks | | ‘Isn’t this fun?’ |
Quotes | | “Isn’t this fun?” |
Dashes | | – is en-dash, — is em-dash |
建立一個自定義清單
- Markdown
- Text-to- HTML conversion tool Authors
- John
- Luke
如何建立一個注腳
一個具有注腳的文本。1
注釋也是必不可少的
Markdown将文本轉換為 HTML。
KaTeX數學公式
您可以使用渲染LaTeX數學表達式 KaTeX:
Gamma公式展示 Γ ( n ) = ( n − 1 ) ! ∀ n ∈ N \Gamma(n) = (n-1)!\quad\forall n\in\mathbb N Γ(n)=(n−1)!∀n∈N 是通過歐拉積分
Γ ( z ) = ∫ 0 ∞ t z − 1 e − t d t . \Gamma(z) = \int_0^\infty t^{z-1}e^{-t}dt\,. Γ(z)=∫0∞tz−1e−tdt.
你可以找到更多關于的資訊 LaTeX 數學表達式here.
新的甘特圖功能,豐富你的文章
- 關于 甘特圖 文法,參考 這兒,
UML 圖表
可以使用UML圖表進行渲染。 Mermaid. 例如下面産生的一個序列圖:
這将産生一個流程圖。:
- 關于 Mermaid 文法,參考 這兒,
FLowchart流程圖
我們依舊會支援flowchart的流程圖:
- 關于 Flowchart流程圖 文法,參考 這兒.
導出與導入
導出
如果你想嘗試使用此編輯器, 你可以在此篇文章任意編輯。當你完成了一篇文章的寫作, 在上方工具欄找到 文章導出 ,生成一個.md檔案或者.html檔案進行本地儲存。
導入
如果你想加載一篇你寫過的.md檔案,在上方工具欄可以選擇導入功能進行對應擴充名的檔案導入,
繼續你的創作。
- 注腳的解釋 ↩︎